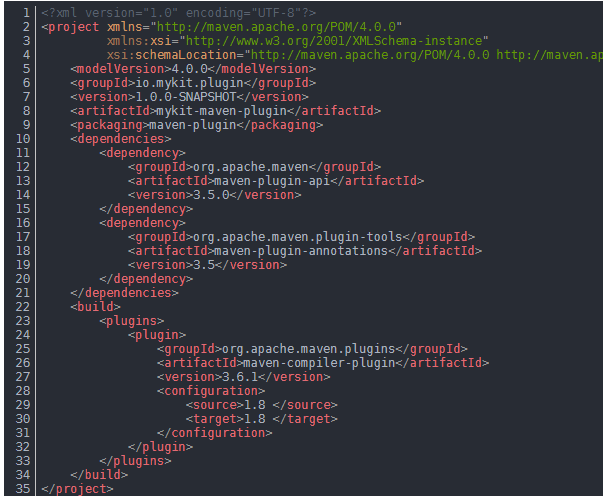
Today, to share a skill that most people will not, that is, we write our own Maven plugin. Well, let’s get straight to today’s topic.
Maven plug-in related concepts
Plugin coordinates positioning
Plugin and ordinary jar package contains the same coordinates positioning attributes that: groupId, artifactId, version, when using the plugin will be searched from the local repository, if not, then from the remote repository to download, such as the following configuration shows.
<! — Uniquely locate the dependency plugin –>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-dependency-plugin</artifactId>
<version>2.10</version>
execution configuration
The execution configuration contains a set of properties that indicate how the plugin will be executed.
id : executor name
phase: identifies the phase of execution
goals: identifies the goal or function of execution
configuration: identifies the configuration file required to execute the goals
Plugin configuration and usage examples
Copy the plugin dependencies to the specified directory.
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-dependency-plugin</artifactId>
<version>3.1.1</version>
<executions
<execution
<id>copy-dependencies</id>
<phase>package</phase>
<goals>
<goal>copy-dependencies</goal
</goals>
<configuration
<outputDirectory>${project.build.directory}/alternateLocation</outputDirectory>
<overWriteReleases>false</overWriteReleases>
<overWriteSnapshots>true</overWriteSnapshots
<excludeTransitive>true</excludeTransitive>
</configuration
</execution
</executions
</plugin>
Use of common plug-ins
Execute the plugin syntax by command
In addition to using plugins via configuration, Maven also provides the ability to invoke plug-in targets directly via commands in the format shown below.
mvn groupId:artifactId:version:goal -D{argument name}
Example of executing a plugin via command
(2) Showing the dependency tree for pom
mvn org.apache.maven.plugins:maven-dependency-plugin:2.10:tree
(3) A direct, simplified version of the command, but only if it is an official maven plugin
mvn dependency:tree
Other common plugins
(1) View the final configuration of the pom file
mvn help:effective-pom
1
(2) Prototype project generation
archetype:generate
(3) Quickly create a web application
mvn archetype:generate -DgroupId=io.mykit.web -DartifactId=mykit-maven-web -DarchetypeArtifactId=maven-archetype-webapp -DinteractiveMode=false
(4) Quickly create a Java project
mvn archetype:generate -DgroupId=io.mykit.java -DartifactId=mykit-maven-java -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
Customizing Maven Plugins
Here, let’s talk about the steps to customize the Maven plugin. Here, I will summarize the steps to customize the Maven plugin as follows.
(1) create a maven plugin project
(2) set the packaging to maven-plugin
(3) add plug-in dependencies
(4) write plug-in implementation logic
(5) package to build plug-ins
Next, let’s implement a custom Maven plugin.
Configure the pom.xml of the plugin project
Here, I directly skipped the creation of the Maven project, I believe that the partners will create Maven projects, here, I will not repeat, directly give the configuration of the pom.xml file, as follows.
<?xml version=”1.0″ encoding=”UTF-8″? >
<project xmlns=”http://maven.apache.org/POM/4.0.0″
xmlns:xsi=”http://www.w3.org/2001/XMLSchema-instance”
xsi:schemaLocation=”http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd”>
<modelVersion>4.0.0</modelVersion>
<groupId>io.mykit.plugin</groupId
<version>1.0.0-SNAPSHOT</version>
<artifactId>mykit-maven-plugin</artifactId
<packaging>maven-plugin</packaging>
<dependencies>
<dependency>
<groupId>org.apache.maven</groupId>
<artifactId>maven-plugin-api</artifactId>
<version>3.5.0</version>
</dependency>
<dependency>
<groupId>org.apache.maven.plugin-tools</groupId>
<artifactId>maven-plugin-annotations</artifactId>
<version>3.5</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.6.1</version>
<configuration>
<source>1.8 </source>
<target>1.8 </target>
</configuration>
</plugin>
</plugins>
</build>
</project>
Maven plugin implementation class
Next, let’s write the implementation class for the Maven plugin. here, I’ll give the source code for the class directly, as follows.
package io.mykit.plugin;
import javafx.beans.DefaultProperty;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin;
import org.apache.maven.plugins.annotations;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
/**
* @author binghe
* @description Custom Maven plugin
*/
@Mojo(name = “binghe”)
public class MykitMavenPlugin extends AbstractMojo {
private final Logger logger = LoggerFactory.getLogger(MykitMavenPlugin.class);
@Parameter(property=”sex”)
String sex;
@Parameter(property=”describe”)
String describe;
public void execute() throws MojoExecutionException, MojoFailureException {
logger.info(String.format(“binghe sex=%s describe=%s”,sex,describe));
}
}
Packaging and installing plugins
Package and install the custom Maven plugins to the local Maven repository as follows.
Package
mvn clean package
Install to the local repository
mvn clean install
Referencing custom plugins in other projects
If you need to use our custom Maven plugins in other projects, just create a Maven project and introduce the following configuration in the Maven project’s pom.xml file.