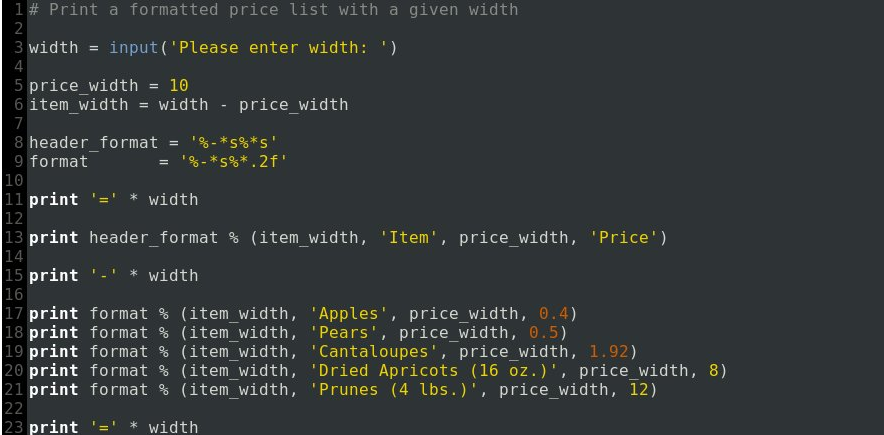
Input and output functions
print()
print() is undoubtedly our most used function, it can output directly, specify interval/end characters, save the output to a specified file (application: logging automation script exceptions), etc. Below is a list of its common uses.
1. Direct output
print(‘hello world’)
output: hello world
2. Specify the interval character sep
print(‘A’, ‘B’, ‘C’, sep=’ Python ‘)
output: A Python B Python C
3. Specify the ending character
print(‘hello’, ‘world’, end=’Python’)
output: hello worldPython
4. Save the output to an outfile.txt file
print(‘hello’, ‘world’, sep=’ ‘, file=open(‘outfile.txt’, ‘w’, encoding=’utf-8′))
input()
input() takes the user input and saves it as a string.
name = input(‘name:’)
The result on jupyter notebook may be different from other editors, but the operation is to press “Enter” after typing.
Get the data type
type()
type() returns the data type of the specified value.
type([1, 2])
output: list
isintance()
isintance() Determines if the value passed in is of the specified type, returns True/False.
isinstance(‘Python New Horizons’, str)
output: True
String operations
str()
str() converts the specified value to a string type.
str(1.23)
output: ‘1.23’
eval()
eval() converts a string into a valid expression to evaluate a value or calculate a result. You can convert strings into lists, tuples, dictionaries, sets, etc.
res = eval(“{‘name’: ‘Python’}”)
type(res)
output: dict
str.capitalize()
capitalize() returns the string with the first letter in upper case and the rest in lower case
cap_str = ‘python new horizons’.capitalize()
cap_str
output: ‘Python New Horizons’
str.center()
center() returns a centered string of the specified width, with the empty left and right parts filled with the specified characters.
width: length
fillchar: the character to fill in the remainder, default is a space
center_str = ‘Python New Horizons’.center(15, “!”)
center_str
output: ‘! !Python New Horizons!!!’
str.count()
str.count(sub, start, end) returns the number of times sub appears in str, you can specify the range by [start, end], if not, the whole string is found by default.
sub: substring
start: the index of the start, default is 0
end: the index of the end, default is the length of the string
name = ‘python python’
# The first time the number of occurrences of ‘p’ is counted by the default range.
# The second time specify start=1, i.e. count from the second character.
name.count(‘p’), name.count(‘p’, 1)
output: (2, 1)
str.find() & str.rfind()
1. find() scans the string from left to right and returns the subscript of the first occurrence of sub. The range can be specified by [start, end], if not, the whole string is found by default. If the string is not found at the end, -1 is returned.
sub: substring
start: the position to start the search, default is 0
end: the end position of the search, default is the length of the string
name = ‘Python’
# The first time the subscript of the first occurrence of ‘Py’ is found in the default range
# Second time specify start=1, i.e., start from the second character.
name.find(‘Py’), name.find(‘Py’, 1)
output: (0, -1)
2. rfind is similar to find(), except that it starts from right to left, i.e. it scans from the end of the string to the beginning of the string.
name = ‘Python’
name.rfind(‘Py’), name.rfind(‘Py’, 1)
output: (0, -1)
str.index() & str.rindex()
1. index() and find() are used in the same way, the only difference is that an error is reported if sub is not found.
Example
name = ‘Python’
name.index(‘Py’, 0)
1
2
output: 0
Example
name = ‘Python’
name.index(‘Py’, 1)
1
2
output: ValueError: substring not found
2. rindex() is used in the same way as index(), but it starts from the right side, and its query is the same as index().
name = ‘Python’
name.rindex(‘Py’, 0)
output: 0
str.isalnum()
isalnum() determines if all characters in the string are letters (can be Chinese characters) or numbers, True, No False, False for an empty string.
Example
‘Python New Horizons’.isalnum()
output: True
Example
‘Python-sun’.isalnum()
output: False
‘-‘ is a symbol, so it returns False.
str.isalpha()
isalpha() determines if all characters in a string are letters (can be Chinese characters), True , No False, False for an empty string.
Example
‘Python New Horizons’.isalpha()
1
output: True
Example
‘123Python’.isalpha()
output: False
which contains a number, returns False
str.isdigit()
isdigit() determines if all characters in the string are digits (Unicode digits, byte digits (single-byte), full-angle digits (double-byte), Roman numerals), Yes True , No False, False for an empty string.
Example
‘four123′.isdigit()
output: False
which contains Chinese digits, return False
Example
b’123’.isdigit()
1
output: True
True for byte numbers.
str.isspace()
String contains only spaces (\n, \r, \f, \t, \v), True, No False, empty string returns False.
Symbol Meaning
\n Line feed
\r carriage return
\f page break
\t horizontal tab
\v vertical tab
‘ \n\r\f\t\v’.isspace()
output: True
str.join()
join(iterable) Merges all the elements of iterable (must be strings) into a new string, using the specified string as separator.
‘,’.join([‘Python’, ‘Java’, ‘C’])
output: ‘Python,Java,C’
str.ljust() & str.rjust()
1. ljust() returns a left-justified string of the specified width
width: length
fillchar: the character to fill in the right margin, the default is a space
ljust_str = ‘Python New Horizons’.ljust(15, “!”)
ljust_str
output: ‘Python New Horizons !!!!!!’
2. rjust() returns a right-justified string of the specified width, the opposite of the ljust operation.
width: length
fillchar: the character to fill in the left-hand margin, the default is a space
rjust_str = ‘Python New Horizons’.rjust(15, “!”)
rjust_str
output: ‘!!!!! !Python New Horizons’
str.lower() & str.islower()
1. lower() Converts the specified string to lowercase.
lower_str = ‘Python New Horizons’.lower()
lower_str
output: ‘python new horizons’
2. islower() determines whether all case-sensitive characters in the string are in lowercase form. yes True , no False, False is returned for an empty string or a string with no case-sensitive characters.
‘python-sun’.islower()
output: True
‘python-sun’ has ‘pythonsun’ as a case-sensitive character and is lowercase, so it returns True.
str.lstrip() & str.rstrip() & str.strip()
1. lstrip() will intercept the left side of the string according to the specified character, if not specified, it will intercept the left side of the empty (space, \r, \n, \t, etc.) part by default.
name = ‘++++Python New Horizons+++’
name.lstrip(‘+’)
output: ‘Python New Horizons ++++’
2. rstrip() is used similarly to lstrip(), except that the right-hand side is intercepted.
name = ‘++++Python New Horizons+++’
name.rstrip(‘+’)
output: ‘++++Python New Horizons’
3. strip() is actually a combination of lstrip() and rstrip(), which will intercept the characters specified on both sides of the string.
name = ‘++++Python New Horizons+++’
name.strip(‘+’)
output: ‘Python New Horizons’
str.split() & str.splitlines()
1. str.split(sep=None, maxsplit=-1) Splits the string using sep as the separator, and returns a list of words in the string.
seq: The separator used to split the string. none (default) means split according to any space, the result will be returned without spaces.
maxsplit: specify the maximum number of splits. -1 (default) means no limit.
split_str = ‘P y t h o n New Perspective’
split_str.split(maxsplit=2)
output: [‘P’, ‘y’, ‘t h o n New Perspective’]
Use default spaces for splitting, set maxsplit to 2
2. str.splitlines returns a list of lines in a string, separated by lines (‘\r’, \n’, ‘\r\n’), and returns the separated list. It has only one argument, keepends, which indicates whether to keep line breaks in the result, False (default) does not keep them, True does.
Example
split_str = ‘P\ny\r t h o n New Perspective’
split_str.splitlines()
output: [‘P’, ‘y’, ‘ t h o n new view’]
Example
split_str = ‘P\ny\r t h o n New Perspective’
split_str.splitlines(keepends=True)
output: [‘P\n’, ‘y\r’, ‘ t h o n New Vision’]
str.startswith() & str.endswith
1. startswith(prefix[, start[, end]]) checks if the string starts with the specified substring substr, Yes True, No False, an empty string will be reported as an error. If start and end are specified, the check is done within the specified range.
startswith_str = ‘Python New Horizons’
startswith_str.startswith(‘athon’, 2)
output: True
Check from the 3rd character
2. str.endswith(suffix[, start[, end]]) is used in the same way as startswith, except that it checks if the string ends with the specified substring, True, False, and an error is reported for an empty string.
endswith_str = ‘Python New Horizons’
endswith_str.endswith(‘athon’, 0, 6)
output: True
Start detecting from the 1st character and end with the 7th character (not including the 7th), note that the range here is actually the same thing as string slicing, both are closed before and open after.
str.title() & str.istitle()
1. title() returns the first letter of each word in the string in uppercase.
title_str = ‘python new horizons python new horizons’.title()
title_str
output: ‘New Horizons in Python New Horizons in Python’
2. istitle() Determine if the string meets the initial capitalization of each word, Yes True , No False, empty string returns False.
‘Abc Def ‘.istitle()
output: True
str.upper() & str.isupper()
1. upper() Converts the letters in the specified string to uppercase.
upper_str = ‘Python New Horizons’.upper()
upper_str
output: ‘PYTHON New Horizons’
2. isupper() determines whether all case-sensitive characters in a string are in uppercase form, True , No False, False for an empty string or a string with no case-sensitive characters.
‘PYTHON-SUN’.isupper()
output: True
List operations
list()
list() transforms an iterable object into a list.
Examples
list((0,1,2)) + list({0,1,2}) + list(‘012’)
output: [0, 1, 2, 0, 1, 2, ‘0’, ‘1’, ‘2’]
Convert a tuple, set, or string into a list and concatenate them with operators.
Example
list(range(3))
output: [0, 1, 2]
Convert an iterable object to a list
list.append()
lst = [‘Python’, ‘Java’]
lst.append(‘C’)
lst
output: [‘Python’, ‘Java’, ‘C’]
list.extend()
extend() extends a list by adding elements from an iterable object (list, tuple, dictionary, string) to the end of the list.
1. append a list
lst = [‘Python’, ‘Java’, ‘C’]
lst.extend([1, 2, 3])
lst
output: [‘Python’, ‘Java’, ‘C’, 1, 2, 3]
2. append the string
lst = [‘Python’, ‘Java’, ‘C’]
lst.extend(‘123’)
lst
output: [‘Python’, ‘Java’, ‘C’, ‘1’, ‘2’, ‘3’]
Append each character of the string as an element to the end of the original list
3. append the set
lst = [‘Python’, ‘Java’, ‘C’]
lst.extend({1,2,3})
lst
output: [‘Python’, ‘Java’, ‘C’, 1, 2, 3]
4. Append the dictionary
lst = [‘Python’, ‘Java’, ‘C’]
lst.extend({1: ‘b’, 2: ‘a’})
lst
output: [‘Python’, ‘Java’, ‘C’, 1, 2]
Append only the key value of the dictionary to the end of the original list
list.insert()
insert(index, object) inserts the specified object into the index position of the list, and shifts the elements of the original index position and elements > index back.
Example
lst = [‘Python’, ‘Java’, ‘C’]
lst.insert(1, ‘C++’)
lst
output: [‘Python’, ‘C++’, ‘Java’, ‘C’]
Example
lst = [‘Python’, ‘Java’, ‘C’]
lst.insert(6, ‘C++’)
lst
output: [‘Python’, ‘Java’, ‘C’, ‘C++’]
When the value of index is greater than the length of the list, it will be added at the end of the list.
list.pop()
pop(index=-1) removes the element at the specified position in the list (the last one by default) and returns the value of the removed element. An error is raised if the specified index value exceeds the length of the list.
lst = [‘Python’, ‘Java’, ‘C’]
lst.pop(1), lst
output:(‘Java’, [‘Python’, ‘C’])
list.remove(element)
lst.remove(value) removes the first occurrence of value from the list, no return value, directly modifies the list; error is reported if value is not in the list.
Example
lst = [‘Python’, ‘Java’, ‘C’, ‘Python’]
lst.remove(‘Python’)
lst
output: [‘Java’, ‘C’, ‘Python’]
Only the first occurrence of Python is removed
example
lst = [‘Python’, ‘Java’, ‘C’, ‘Python’]
lst.remove(‘HTML’)
lst
output: ValueError: list.remove(x): x not in list
HTML is not in the list, error occurred
list.clear()
list.clear() removes all elements of the list, no return value.
lst = [‘Python’, ‘Java’, ‘C’]
lst.clear()
lst
output: []
list.index()
index(value, start, stop) returns the subscript of the first element in the list that matches value, and the range can be specified by [start, stop).
Example
lst = [‘Python’, ‘Java’, ‘C’,
‘Python’, ‘Python’]
lst.index(‘Python’)
output: 0
Find all elements in the list without specifying a range
Example
lst = [‘Python’, ‘Java’, ‘C’,
‘Python’, ‘Python’]
lst.index(‘Python’, 1, 3)
output: ValueError: ‘Python’ is not in list
Specify the range [1, 3), i.e. Python in [‘Java’, ‘C’] obviously does not exist and an error occurs
list.count()
count(value) returns the number of times value appears in the list. If value is not found in the list, 0 is returned.
Example
lst = [‘Python’, ‘Java’, ‘C’,
‘Python’, ‘Python’]
lst.count(‘Python’)
output: 3
Example
lst = [‘Python’, ‘Java’, ‘C’,
‘Python’, ‘Python’]
lst.count(‘Py’)
output: 0
Return 0 if there is no element ‘Py’ in the list.
list.reverse()
reverse() Arrange the list in reverse order, no return value.
lst = [1, 5, 9, 2]
lst.reverse()
lst
output: [2, 9, 5, 1]
list.sort()
sort() sorts the list in the specified way, modifying the original list, which is stable (i.e. the order of two equal elements does not change because of the sort).
key: specify each element of the iterable object to sort in accordance with the function
reverse: False for ascending order, True for descending order.
Example
lst = [1, 5, 9, 2]
lst.sort()
lst
output: [1, 2, 5, 9]
Example
lst = [‘Python’, ‘C’, ‘Java’]
lst.sort(key=lambda x:len(x), reverse=False)
lst
output: [‘C’, ‘Java’, ‘Python’]
Specify key to calculate the length of each element in the list and sort it ascending by length
list.copy()
copy() makes a copy of the list and returns the new list, which is a shallow copy, as explained below.
Example
lst = [[1,2,3], ‘a’ ,’b’]
lst_copy = lst.copy()
lst_copy.pop()
lst, lst_copy
output: ([[1, 2, 3], ‘a’, ‘b’], [[1, 2, 3], ‘a’])
Copy lst and delete the last element of the list lst_copy, the last element of lst is not deleted at this time, which means that the two lists do point to different addresses.
Example
lst = [[1,2,3], ‘a’ ,’b’]
lst_copy = lst.copy()
lst_copy[0].pop()
lst_copy.pop()
lst, lst_copy
output: ([[1, 2], ‘a’, ‘b’], [[1, 2], ‘a’, ‘b’])
Here we perform the same operation as in the previous example, except that this time we delete one more data, i.e. the last element of the nested sublist of the list, and observe the result. This means that the two lists point to different addresses, but the sublists point to the same address.
Extension: direct assignment, shallow copy, deep copy
(1) Direct assignment, just pass the reference of the object. If the original list is changed, the object to which the assignment is made will be changed as well.
(2) shallow copy, no copy subobjects, so the original data subobjects change, the copy of the subobjects will also change.
(3) deep copy, including a copy of the object inside the sub-objects, so the original object changes will not cause any change in the deep copy of the child elements, the two are completely independent.
1. first look at the direct assignment
lst = [1,2,3,[1,2]]
list1 = lst
# — direct assignment —
lst.append(‘a’)
list1[3].append(‘b’)
print(lst,’address:’,id(lst))
print(list1,’address:’,id(list1))
# [1, 2, 3, [1, 2, ‘b’], ‘a’] address: 2112498512768
# [1, 2, 3, [1, 2, ‘b’], ‘a’] Address: 2112498512768
Both lst and list1 will be affected if either of them is changed. 2.
2. A shallow copy requires the copy module, or list.copy(), which has the same effect
from copy import copy
lst = [1, 2, 3, [1, 2]]
list2 = copy(lst)
# —shallow copy–
lst.append(‘a’)
list2[3].append(‘b’)
print(lst,’address:’,id(lst))
print(list2,’address:’,id(list2))
# [1, 2, 3, [1, 2, ‘b’], ‘a’] address: 2112501949184
# [1, 2, 3, [1, 2, ‘b’]] Address: 2112495897728
The addresses of lst and list2 are different, but the addresses of the sublists are still the same, and both will be affected when the elements in the sublists are modified.
3. deepcopy is used in the copy module, where the two lists are completely independent.
from copy import deepcopy
lst = [1, 2, 3, [1, 2]]
list3 = deepcopy(lst)
# — deepcopy —
lst.append(‘a’)
list3[3].append(‘b’)
print(lst,’address:’,id(lst))
print(list3,’address:’,id(list3))
# [1, 2, 3, [1, 2], ‘a’] address: 2112506144192
# [1, 2, 3, [1, 2, ‘b’]] address: 2112499460224
According to the result, you can see that the original list is not changed when the values in the sublist are modified.
Tuple
tuple()
tuple() converts an iterable object into a tuple.
tuple([0,1,2]) + tuple(range(3)) + tuple({0,1,2}) + tuple(‘012’)
output: (0, 1, 2, 0, 1, 2, 0, 1, 2, ‘0’, ‘1’, ‘2’)
Converts iterable objects into lists and concatenates them with operators.
Dictionaries
dict.clear()
clear() Clears the dictionary of all its contents.
dic = {‘CSDN’: ‘Dream丶killer’,
‘Public’: ‘Python New Horizons’}
dic.clear()
dic
output: {}
dict.fromkeys()
fromkeys() creates a new dictionary, with the elements in the sequence iterable as the keys of the dictionary, and value as the initial value corresponding to all keys of the dictionary.
iterable: iterable object, the key of the new dictionary.
value: optional parameter, set the value corresponding to the key sequence, default is None.
dict.fromkeys([‘CSDN’, ‘Public’],
‘Python New Horizons’)
output: {‘CSDN’: ‘Python New Horizons’, ‘public’: ‘Python New Horizons ‘}
dict.get()
get(key, default=None) Find the value of the key according to the specified key, and return the value of the key if it is in the dictionary, otherwise it is None.
Example
dic = {‘CSDN’: ‘Dream丶killer’,
‘Public’: ‘Python New Horizons’}
dic.get(‘CSDN’)
output: ‘Dream丶killer’
Example
dic = {‘CSDN’: ‘Dream丶killer’,
‘Public’: ‘Python New Horizons’}
print(dic.get(‘WeChat’))
output: None
jupyter notebook doesn’t output None by default if you don’t add print, so here we add print to print the result
dict.items()
items() returns a view object, which is a traversable key/value pair that can be converted to a list using list().
Example
dic = {‘CSDN’: ‘Dream丶killer’,
‘Public’: ‘Python New Horizons’}
list(dic.items())
output: [(‘CSDN’, ‘Dream丶killer’), (‘Public’, ‘Python New Horizons’)]
Example
dic = {‘CSDN’: ‘Dream丶killer’,
‘Public’: ‘Python New Horizons’}
for key, value in dic.items():
print(‘key: ‘, key, ‘value: ‘, value)
# key: CSDN value: Dream丶killer
# key: public value: Python New Horizons
dict.keys()
keys() returns a view object with the value of the dictionary’s key, which can be converted to a list.
dic = {‘CSDN’: ‘Dream丶killer’,
‘Public’: ‘Python New Horizons’}
dic.keys()
output: dict_keys([‘CSDN’, ‘Public’])
dict.setdefault()
setdefault(key, default=None) If the key is not in the dictionary, then insert a key with the value None. If the key is in the dictionary, the value of the key is returned.
Example
dic = {‘CSDN’: ‘Dream丶killer’,
‘Public’: ‘Python New Horizons’}
dic.setdefault(‘CSDN’, ‘python-sun’)
output: ‘Dream丶killer’
If the dictionary has the key value CSDN, return the value corresponding to CSDN, no need to insert
Example
dic = {‘CSDN’: ‘Dream丶killer’,
‘Public’: ‘Python New Horizons’}
dic.setdefault(‘microsoft’, ‘python-sun’)
dic
output: {‘CSDN’: ‘Dream丶killer’, ‘Public’: ‘Python New Horizons ‘, ‘weixin’: ‘python-sun’}
If there is no key value for WeChat in the dictionary, we return None, and perform an insertion and assignment according to the set parameter python-sun.
dict.update()
dict.update(dict1) updates the key/value pair of dictionary dict1 to dict, and modifies the value in dict when the key of dict1 appears in dict, or adds the key/value pair if the key does not appear in dict.
dic = {‘CSDN’: ‘Dream丶killer’,
‘Public’: ‘Python New Horizons’}
dic1 = {‘CSDN’: ‘new_name’,
‘weibo’: ‘python-sun’}
dic.update(dic1)
dic
output: {‘CSDN’: ‘new_name’, ‘public’: ‘Python-sun ‘, ‘weibo’: ‘python-sun’}
dict.values()
values() returns a view object with the value of a dictionary, which can be converted to a list.
dic = {‘CSDN’: ‘Dream丶killer’,
‘Public’: ‘Python New Horizons’}
dic.values()
output: dict_values([‘Dream丶killer’, ‘Python New Horizons’])
dict.pop()
pop() removes the key/value of the specified key, and reports an error if the key is not found.
dic = {‘CSDN’: ‘Dream丶killer’,
‘public’: ‘Python New Horizons’}
dic.pop(‘CSDN’)
dic
utput: {‘public’: ‘Python New Horizons’}
dict.popitem()
popitem() removes the end element of the dictionary and returns a tuple of (key, value) pairs. An error is reported if the dictionary is empty.
dic = {‘CSDN’: ‘Dream丶killer’,
‘public’: ‘Python New Horizons’}
dic.popitem()
output:(‘Public’, ‘Python New Horizons’)
Set
set.add()
Adds an element to the set, but does not work if the element already exists in the set.
Example
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set1.add(‘python’)
set1
output: {‘Dream丶Killer’, ‘Python新视野’, ‘python’, ‘python-sun’}
Example
set1 = {‘Dream丶Killer’,
‘Python new horizons’,
‘python-sun’}
set1.add(‘python-sun’)
set1
output: {‘Dream丶Killer’, ‘Python 新视野’, ‘python-sun’}
The added element python-sun is already in the set, so set1 does not change
set.clear()
clear() removes all elements from the set.
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set1.clear()
set1
output: set()
set.difference() & set.difference_update()
1. difference() returns the difference set of multiple sets, which in layman’s terms means which elements of the first set do not appear in the other sets.
Example
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set2 = {‘python’, ‘Dream丶Killer’}
set3 = {‘Python New Horizons’}
set1.difference(set2, set3)
output: {‘python-sun’}
The only element in set1 that does not appear in set2 and set3 is python-sun, so it returns {‘python-sun’} as a set.
Example
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set2 = {‘python’, ‘Dream丶Killer’}
set3 = {‘python new horizons’, ‘python-sun’}
set1.difference(set2, set3)
output: set()
The elements in set1 are all present in set2 and set3, so return the empty set set()
2. The difference_update() method differs from the difference() method in that the difference() method returns a new set with the same elements removed, while the difference_update() method removes the elements of the original set directly, with no return value.
Example
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set2 = {‘python’, ‘Dream丶Killer’}
set3 = {‘Python New Horizons’}
set1.difference_update(set2, set3)
set1
output: {‘python-sun’}
The elements in set1, Dream, Killer and Python New Horizons, both appear in set2 and set3, so these values are removed from set1
Example
set1 = {‘Dream, Killer’,
‘Python New Horizons’,
‘python-sun’}
set2 = {‘python’, ‘Dream丶Killer’}
set3 = {‘python new horizons’, ‘python-sun’}
set1.difference_update(set2, set3)
set1
output: set()
The elements in set1 are all present in set2 and set3, set1 is the empty set after removing all values set()
set.discard()
discard() removes the specified element from the set. If the element specified for removal is not in the set, it is not removed.
Example
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set1.discard(‘python’)
set1
output: {‘Dream丶Killer’, ‘Python新视野’, ‘python-sun’}
If the specified element is not in set1, set1 does not change it
set.intersection() & set.intersection_update()
1. intersection() returns the intersection of sets. If there is no intersection, return the empty set set().
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set2 = {‘python-sun’, ‘Dream丶Killer’}
set3 = {‘python-new-horizons’, ‘python-sun’}
set1.intersection(set2, set3)
output: {‘python-sun’}
Return the elements in set1, set2 and set3 that appear at the same time
2. The difference between the intersection_update() method and the intersection() method is that the intersection() method returns the intersection of the set as the new set, while the intersection_update() method directly modifies the elements of the original set, keeping only the intersection elements, with no return value.
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set2 = {‘python-sun’, ‘Dream丶Killer’}
set3 = {‘python-new-horizons’, ‘python-sun’}
set1.intersection_update(set2, set3)
set1
output: {‘python-sun’}
Only ‘python-sun’ in set1 has appeared in both set2 and set3, so remove the other elements in set1
set.isdisjoint()
isdisjoint() determines if two sets contain the same element, and returns False if they do, or True if they don’t.
Example
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set2 = {‘python-sun’, ‘Dream丶Killer’}
set1.isdisjoint(set2)
output: False
False if there are two identical elements in set1 and set2.
Example
set1 = {‘Dream, Killer’,
‘Python New Horizons’,
‘python-sun’}
set2 = {‘python’}
set1.isdisjoint(set2)
output: True
set1 and set2 have no identical elements, return True
set.issubset()
set2.issubset(set1) Determines if set2 is a subset of set1. Returns True if it is, otherwise returns False.
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set2 = {‘python-sun’, ‘Dream丶Killer’}
set2.issubset(set1)
output: True
set2 is a subset of the set1 set, so it returns True, and you should pay attention to the order of set1 and set2. If written as set1.issubset(set2), it will return False
set.issuperset()
set1.issuperset(set2) determines if the set2 is a subset of the set1 set. It is used in the same way as issubset(), except that the arguments are in opposite positions.
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set2 = {‘python-sun’, ‘Dream丶Killer’}
set1.issuperset(set2)
output: True
set1.pop()
pop() Removes and returns any element of the set. An error is reported if the set is an empty set.
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set1.pop()
output: ‘python-sun’
set.remove()
remove() removes the specified element from the set, or an error occurs if the element is not in the set.
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set1.remove(‘Dream丶Killer’)
set1
output: {‘Python New Horizons’, ‘python-sun’}
set.symmetric_difference()
symmetric_difference() returns the set of non-repeating elements of two sets, i.e. the complement of the two sets, the same as ^.
Example
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set2 = {‘python’, ‘python-sun’, ‘Dream丶Killer’}
set1.symmetric_difference(set2)
output: {‘Python-New Horizons’, ‘python’}
Example
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set2 = {‘python’,
‘python-sun’,
‘Dream丶Killer’}
set1 ^ set2
output: {‘python new horizons’, ‘python’}
The result is the same as above
set.symmetric_difference_update()
set1.symmetric_difference_update(set2) removes the elements of set1 that are identical in set2 and inserts the elements of the set2 set that are different into set1. This simply means that the complement of set1 and set2 is assigned to set1.
Example
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set2 = {‘python’,
‘python-sun’,
‘Dream丶Killer’}
set1.symmetric_difference_update(set2)
set1
output: {‘python new horizons’, ‘python’}
Example
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set2 = {‘python’,
‘python-sun’,
‘Dream丶Killer’}
set1 = set1 ^ set2
set1
output: {‘Python-New Horizons’, ‘python’}
set.union()
union() returns the union of multiple sets. Works the same as |.
Example
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set2 = {‘python’,
‘python-sun’,
‘Dream丶Killer’}
set3 = {‘ABC’}
set1.union(set2, set3)
output: {‘ABC’, ‘Dream丶Killer’, ‘Python New Horizons’, ‘python’, ‘python-sun’}
Example
set1 = {‘Dream丶Killer’,
‘Python new horizons’,
‘python-sun’}
set2 = {‘python’,
‘python-sun’,
‘Dream丶Killer’}
set3 = {‘ABC’}
set1 | set2 | set3
output: {‘ABC’, ‘Dream丶Killer’, ‘Python New Horizons’, ‘python’, ‘python-sun’}
set.update()
update() updates a set using itself and other unions.
Examples
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set1.update([1,2,3])
set1
output: {1, 2, 3, ‘Dream丶Killer’, ‘Python新视野’, ‘python-sun’}
Example
set1 = {‘Dream丶Killer’,
‘Python New Horizons’,
‘python-sun’}
set1 = set1 | set([1,2,3])
set1
output: {1, 2, 3, ‘Dream丶Killer’, ‘Python新视野’, ‘python-sun’}
The same effect can be achieved using |.
For those who have just started Python or want to start Python, you can contact the author through the small card below to exchange and learn together, we all come from a novice, sometimes a simple problem is stuck for a long time, but maybe a little dialing from others will make it clear.