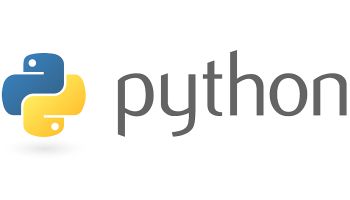
1. Arrange with itertools
In this program, we imported a built-in module called itertools. Using itertools, you can find all permutations of a given string. There are many methods in itertools, you can try combinations and other methods.
2. Single-line conditional expression
This conditional expression has been added to Python 2.5 version. This can be used with the A if condition else B syntax. First, the condition is evaluated and returned based on the Boolean value of the condition. If it is true, return A, otherwise, if it is false, return B.
3. Reverse string
In this program, we use extended slicing to reverse the string, and extended slicing uses the [begin:end:step] syntax. So when we skip the start, end, and step, we pass (-1) as the value. This will reverse the given string.
4. Use Assert to handle exceptions
Exception handling is a very important concept in programming. Use the assert keyword and the given condition to print the error statement. If the given condition is not true, then it will print an error message and terminate the program.
5. Use split on multiple inputs
split() is one of the string methods, it splits the string into a list. The default separator used in this method is a space. In this program, instead of creating three repeated rows for input operations, replace them with one row.
6. Transpose the matrix with zip()
The Zip function has any number of iterable objects from different columns and aggregates the corresponding tuples. The asterisk (*) operator is used to decompress the list. Later the list is changed to the transpose matrix of the given list.
7. Resource Context Manager
Resource management is one of the important tasks in the programming process. Accessing and releasing files, locks, and other resources is a busy task. If the resource is not closed properly, it may cause several problems such as memory leaks. To solve this problem, don’t use the open and close methods every time, but use the context manager shown in the code snippet.
8. Underscore as separator
When using large numbers in a program, use underscores instead of commas as separators to improve readability. Python syntax does not recognize underscores. It is represented by an underscore, represents the number in the preferred format and is readable.
9. Try f string format
The F string format was introduced in Python 3.6. It is the simplest and most feasible method of string formatting. Use the f string format instead of the traditional format to make the code easier to understand.
10. Use this trick to swap integers
Note that swapping integers is done without using temporary variables. Python evaluates expressions from left to right, but in assignment operations, the right side is evaluated first. This creates a tuple for the variables on the right (b and a) whose values are assigned from the variable on the left. This process helps to exchange variables.
11. Use lambda instead of function
Lambda is one of the most powerful functions, also known as anonymous functions. It does not require a name or function definition or return statement. Ordinary functions use the def keyword, while lambda functions use the lambda keyword. It works like a function, except that it only applies to one expression.
12. Print multiple times without loop
In this program, we try to print the statement multiple times using a single line instead of using a loop. The asterisk (*) enables you to print the statement a specified number of times.
13. Unpack the string into a variable
A sequence or a string can be unpacked into different variables. In this program, python string letters will be decompressed into variables separately. The output of the program will be p, y, t.
14. Use Map for List Comprehension
In this program, we try to add elements to the list. For this, we use the lambda function in combination with map and list comprehension. The output of this program will be [12, 15, 18].
15. Remove duplicates from the list
In this program, we try to remove duplicates from the list. One thing to remember is that sets are not allowed to be repeated. We pass the list to set() and change it to a list again, removing all duplicate elements in the list.
16. Conditions in print statements
This program is very interesting and contains quite a few operations. First, the input method will be executed, and then the input value will be changed to an integer. Then it will check the condition and return a boolean value. If it returns, a non-zero number odd number will be output, or if it returns zero, then even number will be output.
17. Condition list All and Any
In this program, we check a list of conditions at a time. There are two functions: all() and any(). As the name implies, when we use all(), all conditions must be true. And when using any(), even if one of the conditions is true, the code block will be executed.
18. Combine two dictionaries
This one is now deprecated. In this program, we try to merge two dictionaries. Please note that in this program, you can use “|” to complete the merge operator.
19. Check the execution time
Check the execution time of the program by importing the timeit package. In this program, the execution time of a list of 1 to 1000 is formed.
20. Check the library
In this program, we try to check the library of functions. All the attributes and modules of itertools will be printed out with this program.